JavaScript Developer Interview Questions
JavaScript, the cornerstone of web interactivity, requires proficient developers to weave dynamic digital experiences. Dive into this guide, which offers critical interview questions tailored to gauge the proficiency of JavaScript developer candidates. Evaluate their expertise in ES6, asynchronous operations, and the intricacies of the JavaScript runtime to ensure dynamic, interactive, and efficient web solutions.
What is JavaScript and where can it be used?
Answer: JavaScript is a versatile, high-level, interpreted programming language primarily used for enhancing web interactivity. Beyond the browser, it's used in servers (Node.js), mobile applications, desktop apps, and more.
2.
Explain the difference between var, let, and const.
Answer: var is function-scoped and hoisted, let is block-scoped and allows reassignment, and const is block-scoped but cannot be reassigned after its initial declaration.
3.
What are JavaScript closures?
Answer: Closures allow a function to access and manipulate variables that are external to that function but exist in its outer function where it was defined.
4.
Describe the concept of hoisting in JavaScript.
Answer: Hoisting is JavaScript's behavior where variable and function declarations are moved to the top of their containing scope during the compile phase, but not their initializations.
5.
How does the this keyword work in JavaScript?
Answer: this refers to the object that's currently executing the current function. Its value depends on how the function was called - whether it's from an object, in a callback, or as a constructor.
6.
What is the difference between == and === in JavaScript?
Answer: == checks for loose equality and performs type coercion if necessary. === checks for strict equality without type coercion.
7.
Explain the event loop in JavaScript.
Answer: The event loop handles asynchronous operations by continually checking the call stack to see if it's empty, then processing any pending events (like API calls) in the message queue.
8.
What are promises in JavaScript?
Answer: Promises are a way to handle asynchronous operations, providing a more flexible way to handle success and error callbacks compared to traditional callback functions.
9.
What is the DOM?
Answer: The Document Object Model (DOM) represents the page so that programs can change the document structure, style, and content dynamically.
10.
How can you handle exceptions in JavaScript?
Answer: Exceptions can be handled using try, catch, finally blocks.
11.
What's the difference between null and undefined?
Answer: undefined means a variable has been declared but not assigned a value. null is an assignment value that represents no value or no object.
12.
What is event delegation in JavaScript?
Answer: Event delegation is the process of handling events at a higher level in the DOM than the element on which the event occurred, utilizing event propagation.
13.
How does prototypal inheritance work in JavaScript?
Answer: In JavaScript, each object has a prototype property, which refers to another object. Objects inherit properties and methods from their prototype.
14.
Explain AJAX in JavaScript.
Answer: AJAX (Asynchronous JavaScript and XML) allows web pages to update asynchronously by fetching data from the server without having to reload the page.
15.
What are arrow functions in JavaScript?
Answer: Arrow functions provide a shorthand syntax for defining functions and do not have their own this, arguments, super, or new.target.
16.
What is destructuring in JavaScript?
Answer: Destructuring allows binding using pattern matching, with the possibility of extracting multiple values from data stored in objects and arrays.
17.
How do you deep clone an object in JavaScript?
Answer: One common way is using JSON.parse(JSON.stringify(object)), but this doesn't handle special objects like RegExp, Map, Set, etc.
18.
Explain the concept of a "callback function".
Answer: A callback function is a function passed into another function as an argument, which is then invoked inside the outer function.
19.
What's the difference between async/await and promises?
Answer: Both handle asynchronous operations, but async/await provides a synchronous-like code style using the async keyword and await expressions, making the code more readable.
20.
What are higher-order functions?
Answer: Higher-order functions are functions that take other functions as arguments, return a function, or both.
21.
How can you prevent default behavior in JavaScript events?
Answer: By using the event.preventDefault() method.
22.
Explain the difference between synchronous and asynchronous code in JavaScript.
Answer: Synchronous code runs sequentially and waits for each operation to complete, whereas asynchronous code doesn't have to wait and can execute subsequent operations in parallel.
23.
What is the spread operator in JavaScript?
Answer: The spread operator (...) is used to split an array into individual elements or combine multiple elements into an array.
24.
How do you ensure code quality in JavaScript?
Answer: Using tools like ESLint or TSLint, writing unit tests, performing code reviews, and following best practices.
25.
Describe the concept of "temporal dead zone" in ES6.
Answer: It's the time span between entering the scope of a variable and the actual variable declaration, where accessing the variable results in a ReferenceError.
Hiring an JavaScript Developers With Braintrust
In your pursuit of JavaScript Developers, we stand ready to assist in finding top talent swiftly. With our services, you can expect to be matched with five highly-qualified JavaScript Developers within just minutes. Let us streamline your recruitment process and connect you with the skilled professionals you seek to meet your needs effectively.
Looking for Work
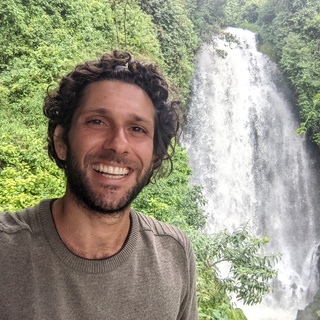
Leland Reardon
Santa Cruz, CA, USA
- Python
- Full Stack Engineering
- JavaScript
- Ruby on Rails
Looking for Work
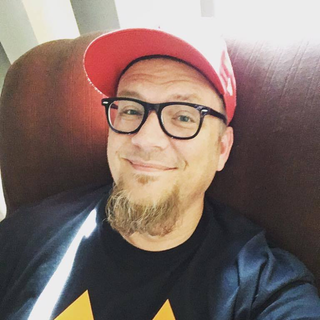
Marcus McBride
Costa Mesa, CA, USA
- React
- JavaScript
- Node.js
Looking for Work
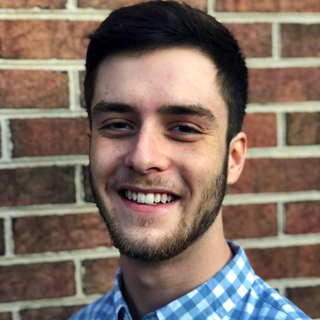
Maxwell Andrew
New York, NY, USA
- React
- Frontend Engineering
- JavaScript
- Ethereum
Get matched with Top JavaScript Developers in minutes 🥳
Hire Top JavaScript Developers